Debug Go Lambda Locally
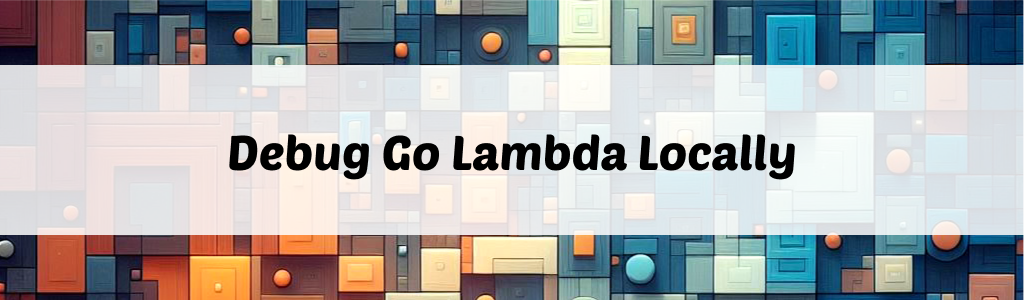
In this short post I’ll show you how to run and debug a Go Lambda function locally without using SAM or Serverless, but just with your IDE and one simple CLI tool that makes RPC requests.
First, before running your Lambda code in debug mode, you need to set _LAMBDA_SERVER_PORT
environment variable. For example, it can be 8080
.
Second, run your Lambda code in debug mode, the same way as you usually run any other Go app you want to debug. It should start and wait to be triggered. You may be tempted to try to trigger your Lambda with curl
, but that won’t work, we’ll need an RPC call.
Third, install awslambdarpc CLI tool by running go install github.com/blmayer/awslambdarpc@latest
for newer versions of Go or go get github.com/blmayer/awslambdarpc
for older versions.
Finally, with _LAMBDA_SERVER_PORT
env var set, with the Lambda running in debug mode, and with awslambdarpc
installed, run the following command to trigger your function:
awslambdarpc -a localhost:8080 -d '{"body": "Hello World!"}'
A real Lambda event (payload) can be quite big, and it’s more convenient to pass it as a file:
awslambdarpc -a localhost:8080 -e event.json
Example event.json:
{
"version": "0",
"id": "f8a990c2-2f93-4713-8b5d-d5b96f35bfd7",
"detail-type": "ECS Task State Change",
"source": "aws.ecs",
"account": "123456789012",
"time": "2016-09-15T21:57:35Z",
"region": "us-east-1",
"resources": ["arn:aws:ecs:us-east-1:123456789012:task/3102878e-4af2-4b3c-b9c1-2556b95b2bbf"],
"detail": {
"clusterArn": "arn:aws:ecs:us-east-1:123456789012:cluster/cluster1",
"containerInstanceArn": "arn:aws:ecs:us-east-1:123456789012:container-instance/04f8c17d-29e0-4711-aa74-852654e477ec",
"containers": [{
"containerArn": "arn:aws:ecs:us-east-1:123456789012:container/40a3b4bd-79ae-4472-a0be-816e5e0044a0",
"lastStatus": "PENDING",
"name": "test",
"taskArn": "arn:aws:ecs:us-east-1:123456789012:task/3102878e-4af2-4b3c-b9c1-2556b95b2bbf"
}],
"createdAt": "2016-09-15T21:30:33.3Z",
"desiredStatus": "RUNNING",
"lastStatus": "PENDING",
"overrides": {
"containerOverrides": [{
"command": ["command1", "command2"],
"environment": [{
"name": "env1",
"value": "value1"
}, {
"name": "env2",
"value": "value2"
}],
"name": "test"
}]
},
"updatedAt": "2016-09-15T21:30:33.3Z",
"taskArn": "arn:aws:ecs:us-east-1:123456789012:task/3102878e-4af2-4b3c-b9c1-2556b95b2bbf",
"taskDefinitionArn": "arn:aws:ecs:us-east-1:123456789012:task-definition/testTD:1",
"version": 1
}
}
That’s it. If you put debugging breakpoints correctly, this is all you need to trigger them and start debugging. Hope this helps!